[Tutorial] How to expand the FSM Controller
Mar 31, 2020 17:06:41 GMT
uberwiggett, tchrisbaker, and 3 more like this
Post by Invector on Mar 31, 2020 17:06:41 GMT
For those who want to go deeper into customization, let's start a conversation on how you can expand the use by creating custom methods, variables, actions, and decisions to have your own specific behavior added without modifying the original scripts.
First, if you don't have an updated version make sure to open the vControlAI script and make it a partial class, like so:
The vIControlAI which is our Interface is already a Partial Interface so that's good to go.
By making it partial, you can now expand this class by creating another script and you will have access to every method and variable from the original vControlAI.
In this example, we're going to create a new example script and add a method for the AI to MoveTo a specific target that can be assign to the inspector:
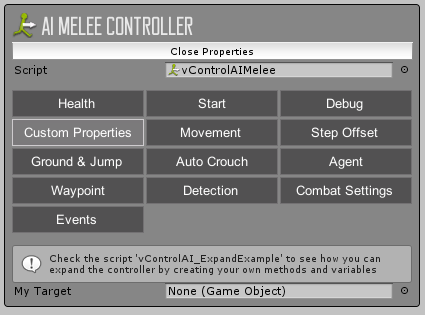
Make sure to save your custom partial script into a personal folder outside the Invector folder, this way you can update the template without losing your content.
To create a new FSM CustomAction open the FSM Window > FSM Component click on the '+' button > Action > New Action Script
(It's the same process to create new custom Decision)

Then simple call your method in the DoAction like this:
And finally for our decision, we access the variable _moveToTarget
Now you can use your new custom method in the FSM Window and create the conditions to play your methods
First, if you don't have an updated version make sure to open the vControlAI script and make it a partial class, like so:
public partial class vControlAI : vAIMotor, vIControlAI
The vIControlAI which is our Interface is already a Partial Interface so that's good to go.
By making it partial, you can now expand this class by creating another script and you will have access to every method and variable from the original vControlAI.
In this example, we're going to create a new example script and add a method for the AI to MoveTo a specific target that can be assign to the inspector:
using UnityEngine;
namespace Invector.vCharacterController.AI
{
/// <summary>
/// This is a simple example on how to expand the AI Controller by creating your own methods, you don't need to modify the core scripts
/// Simple expand the Interface and the Controller here, and you can create new FSM Custom Actions & Decitions to call your custom methods
/// </summary>
// First create a Partial Interface vIControlAI to expand access to the FSM with new methods and variables
public partial interface vIControlAI
{
// Here we created a variable that can be access from my New Action
GameObject customTarget { get; }
bool _moveToTarget { get; }
// Declaring the new Method that will be implemented below
void MoveToTargetExample();
}
// Now create a Partial class vControlAI and implement the new methods and variables from our Interface above
public partial class vControlAI
{
// The vEditorToolbar will display a new tab in your AI Controller Inspector
[vEditorToolbar("Custom Properties")]
// This variable will appear in the new Tab 'Custom Properties'
[vHelpBox("Check the script 'vControlAI_ExpandExample' to see how you can expand the controller by creating your own methods and variables", vHelpBoxAttribute.MessageType.Info)]
public GameObject myTarget;
// Just a bool so we can test the method, check true via inspector to make the controller MoveTo the target assigned
public bool moveToTarget;
// We need to create the bool again so we can pass this information to the FSM
public bool _moveToTarget { get => moveToTarget; }
// This is a simple example for the FSM to have access to the variable myTarget
public GameObject customTarget { get => myTarget; }
// Make it public so you can call it when you create a new FSM CustomAction
// To create a new FSM CustomAction open the FSM Window > FSM Component click on the '+' button > Action > New Action Script
// Then simple call your method in the DoAction like this:
// fsmBehaviour.aiController.MyNewMethod();
public void MoveToTargetExample()
{
// Simple example to move the controller to a specific position set via inspector
if(myTarget != null)
{
// Here we simple call the method MoveTo from the original vControlAI script
MoveTo(myTarget.transform.position);
Debug.Log("Controller Calling 'MoveTo' ");
}
else
{
Debug.Log("Controller Calling 'MoveTo' but a 'myTarget' is not assigned");
}
}
}
}
You can make a whole new core just with this script, add all of your custom methods but you can't override existing methods since it's still the same class, instead copy the method and make the adjustment you need, them call it on your custom action/decision.
You don't need to attach this script to the AI Controller, if you look at the Inspector of your AI the new tab 'Custom Properties' (which is just a name, you can choose any name you want) will be displayed along with your new variables.
You don't need to attach this script to the AI Controller, if you look at the Inspector of your AI the new tab 'Custom Properties' (which is just a name, you can choose any name you want) will be displayed along with your new variables.
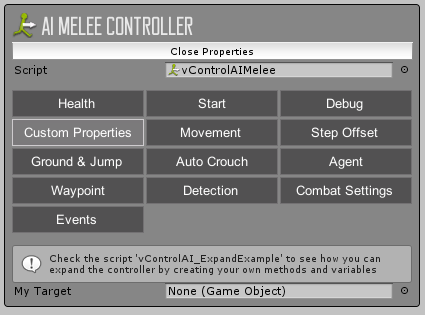
Make sure to save your custom partial script into a personal folder outside the Invector folder, this way you can update the template without losing your content.
To create a new FSM CustomAction open the FSM Window > FSM Component click on the '+' button > Action > New Action Script
(It's the same process to create new custom Decision)

Then simple call your method in the DoAction like this:
using UnityEngine;
namespace Invector.vCharacterController.AI.FSMBehaviour
{
/// <summary>
/// In this example we're calling the method MoveToTargetExample from the <seealso cref="vControlAI_ExpandExample"/>
/// It's just an example on how to expand the AI Controller by adding your own methods and variables
/// </summary>
#if UNITY_EDITOR
[vFSMHelpbox("This is just an example on how to create new Action", UnityEditor.MessageType.Info)]
#endif
public class vMoveToTarget_Example : vStateAction
{
public override string categoryName
{
get { return "Custom Example/"; }
}
public override string defaultName
{
get { return "MoveToTarget Example"; }
}
// set a custom speed to the controller
public vAIMovementSpeed speed = vAIMovementSpeed.Walking;
public override void DoAction(vIFSMBehaviourController fsmBehaviour, vFSMComponentExecutionType executionType = vFSMComponentExecutionType.OnStateUpdate)
{
Debug.Log("FSM Calling 'MoveToTargetExample' ");
fsmBehaviour.aiController.MoveToTargetExample();
fsmBehaviour.aiController.SetSpeed(speed);
}
}
}
And finally for our decision, we access the variable _moveToTarget
using UnityEngine;
namespace Invector.vCharacterController.AI.FSMBehaviour
{
#if UNITY_EDITOR
/// <summary>
/// In this example we're verifying a bool from the script <seealso cref="vControlAI_ExpandExample"/>
/// It's just an example on how to expand the AI Controller by adding your own methods and variables
/// </summary>
[vFSMHelpbox("This is just an example of a custom Decision", UnityEditor.MessageType.Info)]
#endif
public class vCanMoveToTarget_Example : vStateDecision
{
public override string categoryName
{
get { return "Custom Example/"; }
}
public override string defaultName
{
get { return "Can MoveToTarget Example"; }
}
public override bool Decide(vIFSMBehaviourController fsmBehaviour)
{
// This custom decision that will verify the bool 'moveToTarget' and return if it's true or false
return fsmBehaviour.aiController._moveToTarget;
}
}
}
Now you can use your new custom method in the FSM Window and create the conditions to play your methods
